Friday, August 1, 2014
On 12:49 PM by Anonymous
Overview
The accelerated pace of adoption and applications of accelerometers are creating excitement in the engineering and design worlds. Easy to interface via the I2C bus, and very sensitive, an accelerometer measures tilt and motion. They are commonly used in portable devices and game controllers to detect orientation.In this tutorial we will demonstrate how to interface the ADXL345 accelerometer to the BeagleBone Black and display, in 3D, the sensor results. We will use Python, GnuPlot and Debian in this tutorial. Let's take a look at these steps together.
Special thanks to contributing author: David St-Pierre
What You Will Need
- BeagleBone Black
- BeagleBone expandable case Orange or Black (recommended)
- Mini Breadboard
- Jumper Wire Pack for Breadboard
- AC adapter 5 Volts, 2 Amp (suggested)
- Debian Linux
- ADXL345 Accelerometer from Analog Devices available at MPJA
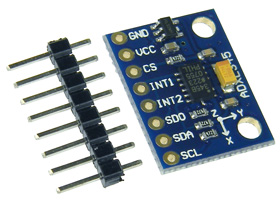


Introduction
In this project, we will interface an ADXL345 3-Axis Accelerometer to the BeagleBone Black. A python program will communicate over the digital I2C Bus and collect X-Y-Z data from the sensor. The data will be stored in a file. The data file will then be plotted in 2D showing the X,Y,Z motion and activity during the recorded period. A 3D plot will then be generated representing the 3D travel of the sensor.Instructions
Connections to the accelerometer can be made with a solderless breadboard and a jumper wire kit.STEP 1 - Circuit Connections
Turn OFF the BeagleBone Black power and make the following connections.Interfacing to the sensor is simple. Only 4 connections are required.
Device Pin | BeagleBone | Signal |
---|---|---|
GND | P9-1 | GND |
VCC | P9-3 | +3.3V |
SDA | P9-20 | I2C2-SDA |
SCL | P9-19 | I2C2-SCL |
STEP 2 - Required Software
Download Gnuplotsudo apt-get update && upgrade sudo apt-get install gnuplot sudo apt-get install gnuplot-x11
You may also need to download VNC (if you have not previously done so)
sudo apt-get install x11vnc
Download ADXL345 pythonLibrary File (modified for BeagleBone Black)
STEP 3 - Program Listing
This is a python program, that interfaces with the ADXL345 library, which has been adapted for the BeagleBone Black.Download getAccel.py File (and save it without the txt extension)
#!/bin/python from adxl345 import ADXL345 from time import sleep adxl345 = ADXL345() myfilename = "accel.dat" with open(myfilename,'w') as f: print"ADXL345 on address 0x%x:" % (adxl345.address) f.write(str("#X,Y,Z ADXL345 accelerometer readings\n")) try: for i in range(1000): axes = adxl345.getAxes(True) xVal = axes['x'] yVal = axes['y'] zVal = axes['z'] print "x = %.3fG" % ( xVal ) print "y = %.3fG" % ( yVal ) print "z = %.3fG" % ( zVal ) f.write(str(xVal) + ",") f.write(str(yVal) + ",") f.write(str(zVal) + '\n') sleep(.1) except KeyboardInterrupt: exit()
STEP 4 - 2D Plotting
This is a gnuPlot program that takes the collected sensor data and plots in 2D the X,Y and Z values measured by the accelerometer.Download graphData.gp File (and save it without the txt extension)
#!/usr/bin/gnuplot -persist #Author:David St.Pierre #Date:July 31, 2014 reset set yrange[-2:2] set datafile separator "," set mytics 10 set title "ADXL345 2D Plot" set xlabel "Time (0.1 secs)" set ylabel "Gs" set grid plot 'accel.dat' using 0:1 with lines lt 1 title "X axis", 'accel.dat' using 0:2 with lines lt 2 title"Y axis", 'accel.dat' using 0:3 with lines lt 3 title"Z axis" set terminal png set output "myAccelData2d.png" replot pause -1 "Heres your graph\n\nPress enter..." # EOF
STEP 5 - 3D Plotting
This is a gnuPlot program that takes the collected sensor data and plots in 3D the X,Y and Z values measured by the accelerometer.Download graphData3d.gp File (and save it without the txt extension)
#!/usr/bin/gnuplot -persist # # # G N U P L O T # Version 4.6 patchlevel 0 last modified 2012-03-04 # Build System: Linux armv7l # # Copyright (C) 1986-1993, 1998, 2004, 2007-2012 # Thomas Williams, Colin Kelley and many others # # gnuplot home: http://www.gnuplot.info # faq, bugs, etc: type "help FAQ" # immediate help: type "help" (plot window: hit 'h') # set terminal wxt 0 # set output reset unset clip points set clip one unset clip two set bar 1.000000 front set border 31 front linetype -1 linewidth 1.000 set timefmt z "%d/%m/%y,%H:%M" set zdata set timefmt y "%d/%m/%y,%H:%M" set ydata set timefmt x "%d/%m/%y,%H:%M" set xdata set timefmt cb "%d/%m/%y,%H:%M" set timefmt y2 "%d/%m/%y,%H:%M" set y2data set timefmt x2 "%d/%m/%y,%H:%M" set x2data set boxwidth set style fill empty border set style rectangle back fc lt -3 fillstyle solid 1.00 border lt -1 set style circle radius graph 0.02, first 0, 0 set style ellipse size graph 0.05, 0.03, first 0 angle 0 units xy set dummy x,y set format x "% g" set format y "% g" set format x2 "% g" set format y2 "% g" set format z "% g" set format cb "% g" set format r "% g" set angles radians set grid nopolar set grid xtics nomxtics ytics nomytics noztics nomztics \ nox2tics nomx2tics noy2tics nomy2tics nocbtics nomcbtics set grid layerdefault linetype 0 linewidth 1.000, linetype 0 linewidth 1.000 set raxis set key title "" set key inside right top vertical Right noreverse enhanced autotitles nobox set key noinvert samplen 4 spacing 1 width 0 height 0 set key maxcolumns 0 maxrows 0 set key noopaque unset label unset arrow set style increment default unset style line unset style arrow set style histogram clustered gap 2 title offset character 0, 0, 0 unset logscale set offsets 0, 0, 0, 0 set pointsize 1 set pointintervalbox 1 set encoding default unset polar unset parametric unset decimalsign set view 60, 30, 1, 1 set samples 100, 100 set isosamples 10, 10 set surface unset contour set clabel '%8.3g' set mapping cartesian set datafile separator "," unset hidden3d set cntrparam order 4 set cntrparam linear set cntrparam levels auto 5 set cntrparam points 5 set size ratio 0 1,1 set origin 0,0 set style data points set style function lines set xzeroaxis linetype -2 linewidth 1.000 set yzeroaxis linetype -2 linewidth 1.000 set zzeroaxis linetype -2 linewidth 1.000 set x2zeroaxis linetype -2 linewidth 1.000 set y2zeroaxis linetype -2 linewidth 1.000 set ticslevel 0.5 set mxtics default set mytics default set mztics default set mx2tics default set my2tics default set mcbtics default set xtics border in scale 1,0.5 mirror norotate offset character 0, 0, 0 autojustify set xtics autofreq norangelimit set ytics border in scale 1,0.5 mirror norotate offset character 0, 0, 0 autojustify set ytics autofreq norangelimit set ztics border in scale 1,0.5 nomirror norotate offset character 0, 0, 0 autojustify set ztics autofreq norangelimit set nox2tics set noy2tics set cbtics border in scale 1,0.5 mirror norotate offset character 0, 0, 0 autojustify set cbtics autofreq norangelimit set rtics axis in scale 1,0.5 nomirror norotate offset character 0, 0, 0 autojustify set rtics autofreq norangelimit set title "" set title offset character 0, 0, 0 font "" norotate set timestamp bottom set timestamp "" set timestamp offset character 0, 0, 0 font "" norotate set rrange [ * : * ] noreverse nowriteback set trange [ * : * ] noreverse nowriteback set urange [ * : * ] noreverse nowriteback set vrange [ * : * ] noreverse nowriteback set xlabel "" set xlabel offset character 0, 0, 0 font "" textcolor lt -1 norotate set x2label "" set x2label offset character 0, 0, 0 font "" textcolor lt -1 norotate set xrange [ -2 : 2 ] noreverse nowriteback set x2range [ * : * ] noreverse nowriteback set ylabel "" set ylabel offset character 0, 0, 0 font "" textcolor lt -1 rotate by -270 set y2label "" set y2label offset character 0, 0, 0 font "" textcolor lt -1 rotate by -270 set yrange [ -2 : 2 ] noreverse nowriteback set y2range [ * : * ] noreverse nowriteback set zlabel "" set zlabel offset character 0, 0, 0 font "" textcolor lt -1 norotate set zrange [ -2 : 2 ] noreverse nowriteback set cblabel "" set cblabel offset character 0, 0, 0 font "" textcolor lt -1 rotate by -270 set cbrange [ * : * ] noreverse nowriteback set zero 1e-08 set lmargin -1 set bmargin -1 set rmargin -1 set tmargin -1 set locale "en_US.UTF-8" set pm3d explicit at s set pm3d scansautomatic set pm3d interpolate 1,1 flush begin noftriangles nohidden3d corners2color mean set palette positive nops_allcF maxcolors 0 gamma 1.5 color model RGB set palette rgbformulae 7, 5, 15 set colorbox default set colorbox vertical origin screen 0.9, 0.2, 0 size screen 0.05, 0.6, 0 front bdefault set style boxplot candles range 1.50 outliers pt 7 separation 1 labels auto unsorted set loadpath set fontpath set psdir set fit noerrorvariables GNUTERM = "wxt" splot 'accel.dat' u 1:2:3 with lines lt 1 title"3Axis in 3d" pause -1 "Heres your graph\n\nPress enter..." # EOF
STEP 6 - Auto Measure and Plotting
Finally, we put everything together in a single file.Create this file using:
nano autoPlot.sh
and enter the following:
#!/bin/bash sudo python getAccel.py ./graphData.gp ./graphData3d.gp
Cntl+o, (enter), Cntl+x to write and exit the editor.
This bash file calls the getAccel.py program that acquires the sensor data, until Cntl+C is pressed. Then call the gnuPlot programs to plot the accelerometer data in 2D and next in 3D.
Before we can run this file, we need to make the scripts executable.
chmod a+x graphData.gp chmod a+x graphData3d.gp chmod a+x autoPlot.sh
STEP 7 - Running the Program
Start this program and move the sensor in the air (creating acceleration data).This command will start collecting sensor data until you press Cntl+c, then plot the results.
In order to see the graphical output (graphs) you must access the BeagleBone via a VNC session, or directly connected to a monitor and keyboard.
./autoPlot.sh
Sample 2D Plot - Not Moving
Z-Axis is showing Earth gravity (acceleration 9.8m/s^2)
Sample 3D Plot - Not Moving
Single (tiny red) dot in Space (sensor not moving)
Sample 2D Plot - Spinning on itself
Sensor rotating on Axis (note shift in rotation/waves)
Sample 3D Plot - Spinning on itself
Free Spinning in space (hand-held)